本文写于:2016年9月22日 13:25:29,如果时间久远部分细节可能存在变动,请访问相关网站获取最新信息
Laravel 致力于让整个 PHP 开发过程变得让人愉悦,包括本地开发环境,为此官方为我们提供了一整套本地开发环境 —— Laravel Homestead。
Laravel Homestead 是一个打包好各种 Laravel 开发所需要的工具及环境的 Vagrant 盒子(Vagrant 提供了一个便捷的方式来管理和设置虚拟机),该盒子为我们提供了优秀的开发环境,有了它,我们不再需要在本地环境安装 PHP、HHVM、Web服务器以及其它工具软件,我们也完全不用再担心误操作搞乱操作系统 —— 因为 Vagrant 盒子是一次性的,如果出现错误,可以在数分钟内销毁并重新创建该 Vagrant 盒子!
Homestead可以运行在 Windows、Mac 以及 Linux 系统上,其中已经安装好了Nginx、PHP7.0、MySQL、Postgres、Redis、Memcached、Node以及很多其它开发 Laravel 应用所需要的东西。
如果你使用的是Windows,需要开启系统的硬件虚拟化(VT-x),这通常可以通过BIOS来开启。
1. 安装VirtualBox
下载地址:https://www.virtualbox.org/wiki/Downloads
安装过程中一路下一步就可以了
2. 安装Vagrant和Laravel盒子
下载地址:https://www.vagrantup.com/downloads.html
安装过程没有什么特殊操作,别忘了在安装后重启操作系统
重启完成之后,可以通过下面的命令来查看安装是否成功
vagrant --version
然后我们使用下面的命令下载Laravel Homestead Vagrant盒子
vagrant box add laravel/homestead
这一步会花费很长时间,部分地区可能还会被墙,可以使用VPN或者把curl.exe加入你的代理来解决(比如使用Proxifier搭配SS)
3. 安装PHP
我们到:http://windows.php.net/download 下载对应的PHP版本,我使用的是php-7.0.10-nts-Win32-VC14-x64.zip
PHP高版本可能需要安装对应的VC库,这里给出下载链接
VC11:https://www.microsoft.com/zh-CN/download/details.aspx?id=30679
VC14:https://www.microsoft.com/zh-CN/download/details.aspx?id=48145
一般我们把X86和X64的全部安装
把下载下来的PHP解压到你想要的位置,比如D:\php,然后别忘了把这个路径添加到你的用户环境变量里。完成后,重开cmd窗口输入php -v 应该可以显示你安装的php版本
然后我们进入刚才的php安装目录,拷贝php.ini-development 重命名为php.ini ,然后打开编辑,用查找找到下面的行,将其前面的分号都去掉,然后保存。
extension_dir = "ext"
extension=php_openssl.dll
extension=php_mbstring.dll
4. 安装nodejs
下载地址:https://nodejs.org/en/download/
找到对应的安装包下载安装就可以了,安装完成后可以在cmd中使用node -v 来检测是否安装成功。
然后我们全局安装gulp
npm install -g gulp
5. 安装Composer
下载地址:https://getcomposer.org/download/
安装过程中如果php路径没有显示请检查你的环境变量,如果出现SSL没有启动的问题请检查你是否参照上文修改了php.ini中extension的配置。
安装完成后在cmd输入composer –version 来检查是否安装成功。
然后,我们把修改composer的源到composer中国镜像(http://pkg.phpcomposer.com/),加快速度。修改方式是打开cmd执行下面的命令
composer config -g repo.packagist composer https://packagist.phpcomposer.com
6. 安装Git
下载地址:https://git-scm.com/downloads
安装过程中请注意在下面的步骤中
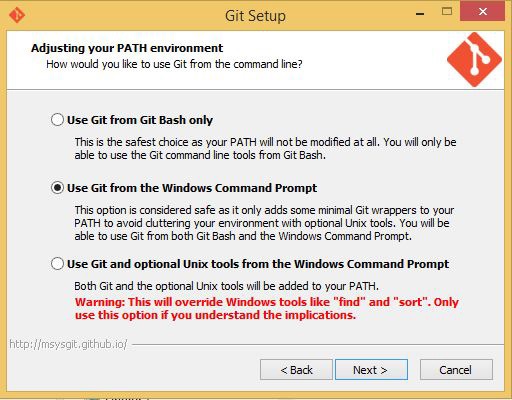
确保选择Use Git from the Windows Command Prompt选项,其他步骤下一步即可。
安装完成后在cmd输入git –version 来检查是否安装成功。
在 Windows 开始菜单找到 Git Bash 并运行,在打开的窗口中执行下面命令,一路回车使用默认选项即可,这将生成SSH Key。(别忘了修改你的email地址)
ssh-keygen -t rsa -C "[email protected]"
7. 安装laravel Install
打开cmd运行下面的命令
composer global require "laravel/installer"
8. 安装Laravel 5.3
打开你准备放置Laravel 5.3 RC1项目的文件夹,在当前文件夹运行cmd来安装laravel(可能需要科学上网)
laravel new demo
完成后,你的项目将被安装到demo文件夹下。我们使用cd demo 来进入demo目录
接着,我们安装所有的依赖
npm install
注意:从这一步开始我们后面的cmd运行目录均为demo文件夹下
9. 安装homestead
在cmd中运行下面指令安装homestead,我这里采用的是项目局部安装
composer require laravel/homestead --dev
执行完后,执行下面命令生成Homestead.yaml
vendor\bin\homestead make
生成之后的内容大概如下
---
ip: "192.168.10.10"
memory: 2048
cpus: 1
hostname: demo
name: demo
provider: virtualbox
authorize: ~/.ssh/id_rsa.pub
keys:
- ~/.ssh/id_rsa
folders:
- map: "C:/Users/XXX/PhpstormProjects/demo"
to: "/home/vagrant/demo"
sites:
- map: homestead.app
to: "/home/vagrant/demo/public"
databases:
- homestead
# blackfire:
# - id: foo
# token: bar
# client-id: foo
# client-token: bar
# ports:
# - send: 50000
# to: 5000
# - send: 7777
# to: 777
# protocol: udp
在这个文件中,我们的虚拟机IP是192.168.10.10。
10. 启动Vagrant,所有步骤完成
我们在cmd运行vagrant up 来启动虚拟机,这一步骤的首次运行可能时间较长。
启动过程中可以观察到端口转发,我们本地的127.0.0.1的8000端口被转发到了192.168.10.10的80端口。
启动完成后我们ping一下192.168.10.10,如果不通的话请打开“控制面板\网络和 Internet\网络和共享中心”,就是适配器管理这个页面,找到最新添加的VM Box Host Only Network。如何判断最新呢,就是根据最后面#后面的数字。这里面是#2这个
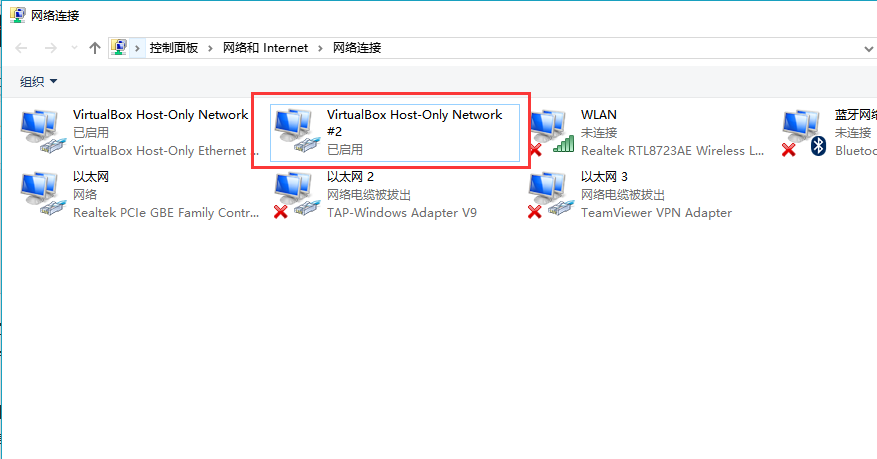
右键进入IPV4设置,修改如下
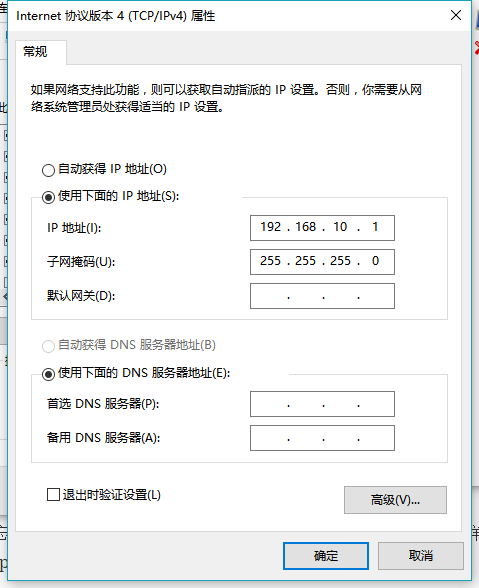
确认之后再ping,ping通后我建议你再运行一下vagrant halt 和vagrant up ,重启虚拟机。
最后别忘了在hosts文件中添加下面的内容,把域名指向虚拟机IP,这样以后我们就可以通过http://homestead.app 来访问我们的项目了
192.168.10.10 homestead.app
运行完后,我们打开http://homestead.app/ 就可以看到我们的laravel了
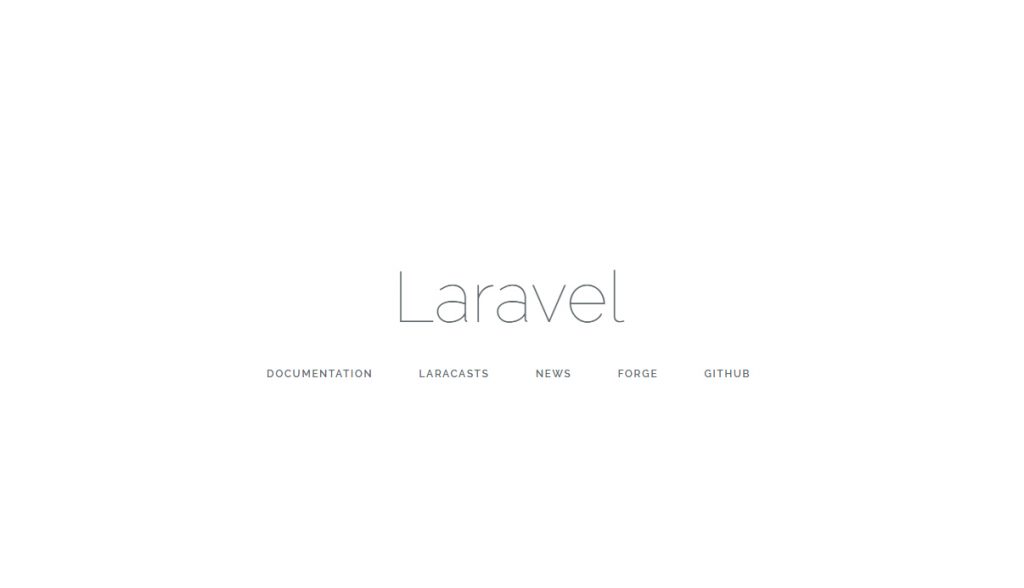
注意:即使关闭当前cmd窗口虚拟机也不会关闭,想关闭虚拟机请打开cmd运行vagrant halt